Linked List Iterator C++
Why Std Binary Search On Std List Works In C Stl By Ashish Patel Codebrace Medium

Iterator Pattern Wikipedia

A Doubly Linked List Prevnextdata There S The Need To Access A List In Reverse Order Header Dnode Ppt Download

Arraylist Vs Linkedlist Vs Vector

Arraylist Vs Linkedlist Vs Vector Dzone Java
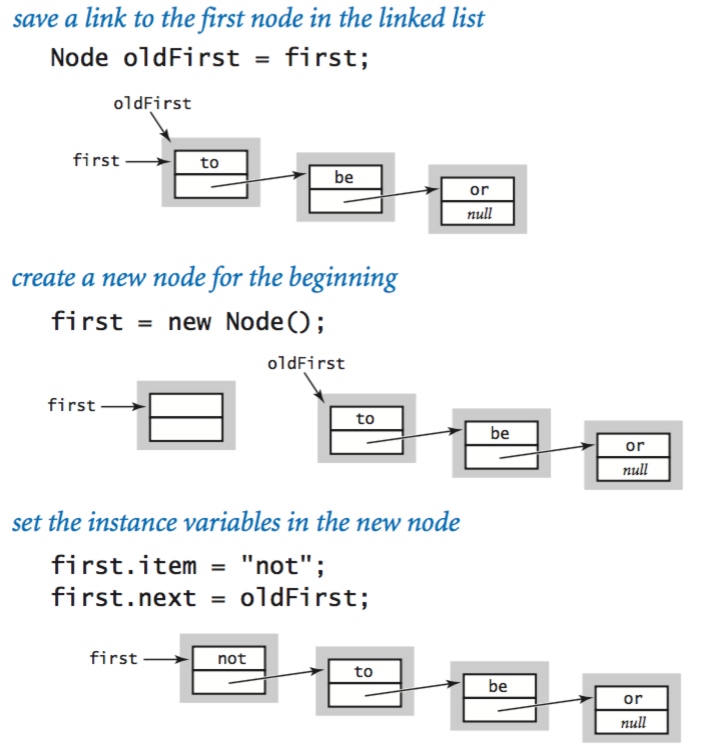
Stacks And Queues
Using difference_type = int;.
Linked list iterator c++. I'm not aware of any exceptions to this, but it's possible since the standard doesn't require specific implementations. This is how the program will traverse the linked list. The main advantage of linked lists over arrays is that the links provide us with the capability to rearrange the item efficiently.
This function was introduced in Qt 5.14. Iterating through list in Reverse Order using reverse_iterator. A node has two parts:.
The linked list is an ordered list of elements, we call them as node, where each node holds the address of its successor node. In this post, we are going to implement the iterator for doubly linked list in java. For example, suppose the linked list contains three nodes that hold value 1,2 and 3.
Add an extra pointer to each node, pointing at the previous node. In the last post we learnt how to traverse a LinkedList using ListIterator. Pointers (without pointer arithmetic) can also be used to iterate through some non-sequential structures.
The next pointer of the tail node is set to NULL as this is the last node. They are slower than other standard sequence containers (arrays, vectors) for retrieval of any item. Doubly Linked In C++.
Here we will learn how to iterate a LinkedList using Iterator. Iterator design pattern works on Iterator point. Extra memory space for a pointer is required with each element of the list.
The C++ doubly linked list has nodes that can point towards both the next and the previous node. The ordering is kept internally by the association to each element of a link to the element preceding it and a link to the element following it. The singly-linked list is the easiest of the linked list, which has one link per node.
A single-linked list is represented by a pointer to the first node of the linked list. Adding, removing and moving the elements within the list or. It accepts 2 arguments, the container and iterator to position where the elements have to be inserted.
C++ std::list is usually implemented as a doubly linked list. An Iterator can be used to loop through an LinkedList. In the previous post, we made a linked list using the code given below.
Implementing Iterator pattern of a single Linked List. Now let's look at two other major features of this implementation:. // Get Descending List Iterator from List Object Iterator<String> listItReverse = listOfStr.descendingIterator();.
One possibility is writing a doubly-linked list class:. In this tutorial you will learn about doubly linked list in C and C++. It is implemented as a singly-linked list.
C++11 mercredi 6 mai. Intermediate C++ 18 -- Linked Lists, Iterator. Linked lists in C++ (Singly linked list) Linked list traversal using while loop and recursion in C++;.
#include <algorithm> struct Player { int id;. What is the using keyword?. 4 The position of new iterator using prev() is :.
What is Linked List?. To create linked list in C/C++ we must have a clear understanding about pointer. Doubly linked lists do not suffer from the awkward special-casing issues associated with singly linked lists.
Linked list is a linear data structure in which elements are linked using pointers. In this tutorial we will understand the working of Doubly Linked List & see all operations of Doubly Linked List. DoubleLinkedList(C++ Code) An implementation of a doubly linked list in C++, with template and iterator.
Here now is the full source code for our complete singly linked list class. The data part and the next part. Furthermore, we will also declare these iterators into the linked list class itself (publically).
Lists are sequence containers which are implemented as doubly linked list & allows iteration in both directions and takes O(1) time for insertion and deletion. But we can’t directly call the erase() method inside a for loop since calling it invalidates the iterator. Then, you can define additional operators on your iterators like --, and maybe even better, you can make insert_before and remove simple and efficient.
Contribute to deadlift1226/Linked-List-Iterator development by creating an account on GitHub. The iterator for the list class is defined as an inner class in the list class, and stores a pointer to the node before the node that we want the iterator to point to. The front node is known as head node and next of last node is null.
One of the key aspects of Software Engineering is to avoid reinventing the wheel. Iterator Pattern – Design Patterns (ep 16) - Duration:. Linked Lists A linked list is a basic data structure where each item contains the information that we need to get to the next item.
Std::list is a container that supports constant time insertion and removal of elements from anywhere in the container. Compared to std::forward_list this container provides bidirectional iteration capability while being less space efficient. We can handle this in many ways:.
To Implement the iterator for singly linked list, we should provide the implementation for hashNext () and next () method. In this C++ tutorial, we are going to discuss how to search an element in a singly Linked list in C++. As in the singly linked list, the doubly linked list also has a head and a tail.
What is a Linked List in C++?. Std::forward_list is a container that supports fast insertion and removal of elements from anywhere in the container. Although the code was initially developed in "C" before the days of C++, the code worked and performed quite well.
To allow assignment of iterators to const_iterators (but not the other way around) we will create a heirarchy where const_iterator is the base class and iterator is the derived class. Unlike arrays, elements are not stored in a contiguous memory location. In looking at the details of the Hewlett-Packard STL list class source code, we've gone over the class data and function members and some of the type definitions inside the class.
This class features the use of a dummy head node, so that even empty lists will contain at least one node. The first node is called head. A singly-linked list and a doubly-linked list.
An iterator in a doubly linked list is bidirectional-- we can move backwards or forwards in a doubly linked list. Linked list elements are not stored at contiguous location;. The previous pointer of the head is set to NULL as this is the first node.
The idea is to iterate the list using iterators and call list::erase on the desired elements. There are two types of linked lists:. If the linked list is empty, then value of head is NULL.
The singly-linked list contains nodes that only point to the next node. Iterator<T> *getIteratorAtTail() { Iterator<T> *i = new Iterator<T>(tail);. Iterators 1 Double Linked and Circular Lists node UML diagram implementing a double linked list the need for a deep copy 2 Iterators on List nested classes for iterator function objects MCS 360 Lecture 12 Introduction to Data Structures Jan Verschelde, 10 February Introduction to Data Structures (MCS 360) Iterators L-12 10 February 1/31.
It is usually implemented as a doubly-linked list. Compared to std::list this container provides more space efficient storage when bidirectional iteration is not needed. Initially, I possessed what I thought was a pretty good set of doubly linked list routines.
Using insert and the function end, the functionality of push_back, which adds an element to the end of the list, could also be implemented as. Fast random access is not supported. Make sure that you are familiar with the concepts explained in the post(s) mentioned above before proceeding further.
The position of new iterator using next() is :. QLinkedList (std::initializer_list < T > list) Constructs a list from the std::initializer_list specified by list. Player(int playerId, std::string playerName) :.
Singly linked list is a type of data structure that is made up of nodes that are created using self referential structures. List containers are implemented as doubly-linked lists;. ####Compile the test file and try it g++ -o test_list test.cpp.
Now I will explain in brief what is pointer and how it works. Intermediate C++ 18 -- Linked Lists, Iterator - Duration:. Representing Linked Lists in C++.
Each of these nodes contain two parts, namely the data and the reference to the next list node. Also, hasNext () will tell if previous element is available or not i.e. In singly linked list, we can move/traverse only in one single direction because each node has the address of the next node only.Suppose we are in the middle of the linked list and we want the address of previous node then we don’t have any option other than repeating the traversing from the beginning node.
} This method will just return a pointer to an iterator initiated at the tail of the list. Implementing an iterator class for a my linked list. The elements are linked using pointers.
These had evolved over time and had been tested on so many operating systems that I was satisfied that my code was fast, robust, and bug-free. Visual Studio implements std::list as a circular doubly linked list with a dummy node. Adding, removing and moving the elements within the list, or across several.
A C++ implementation of a node is quite simple:. It makes life lot easier, especially when your focus is on problem solving and you don’t want to spend time in implementing something that is already available which guarantees a robust solution. Note that the STL list container supports iterating both forward and in reverse (because it is implemented as a doubly linked list).
Fast random access is not supported. Concatenating two linked lists in C++;. Std::list::end is an iterator to the dummy node.
We can iterate through the list by following the chain of pointers. Rob Edwards from San Diego State University instills how to iterate through a linked list and get all the data. In previous post, we have already seen the iterator for singly linked list.The basic difference between iterators of singly and doubly linked list is, in case of singly linked list, we can iterator in forward direction only but in case of doubly linked list, we can iterate in both forward and backward directions.
The cost is that the list takes more space in memory. And that’s all for the classes that will handle our doubly linked list with class templates. Now calling function next () with descending iterator will return the previous element and also moves the cursor by one position backward.
Constructs a list with the contents in the iterator range first, last). Print elements of a linked list in forward and reverse order using. Each node in a list consists of at least two parts:.
As such, they are useful as general purpose classes, which is why the. Using value_type = T;. STL is one of the pillars of C++.
The conductor will be a pointer to node, and it will first point to root, and then, if the root's pointer to the next node is pointing to something, the "conductor" (not a technical term) will be set to point to the next node. Understand this word non-contigous,this means that the elements are stored in random and non consecutive locations unlike an array so they are linked using pointers.While adding any new element we maintain a continous link so as to traverse through them later.Below is the implementation. In this post, we will see how to print a std::list in C++.
Internally, JAVA calls these methods to check the availability of data and fetch the next data from any linear collections if class implements Iterable. I believe this is relatively new that an iterator class is required to have the following tags at the top of the class:. Linked lists in C++ (Singly linked list) Make sure that you are familiar with the concepts explained in the post(s) mentioned above before proceeding further.
Doubly Linked list is a type of Linked List Data structure which behaves like a two way list/chain.The reason it is called a two way list or two way chain is because we can traverse this list in two. In a linked list, each element is connected to the prior element by a pointer. In this fashion, the list can be traversed.
The method hasNext( ) returns true if there are more elements in LinkedList and false otherwise. STL singly linked lists (revision 3) The standard container class std::list is a doubly linked list. We will proceed further by taking the linked list we made in the previous post.
You can make a supplement to it. ''' using iterator_category = std::bidirectional_iterator_tag;. A basic layout of the doubly linked list is shown in the below diagram.
Each node will hold values and the address of the next node. A pointer is a variable that contains the address of a variable. The value type of InputIterator must be convertible to T.
Inserter() :- This function is used to insert the elements at any position in the container. If you don’t know what a Linked List Data Structure is please check this post. Std::list is a standalone class.

It C Linked List Simulation
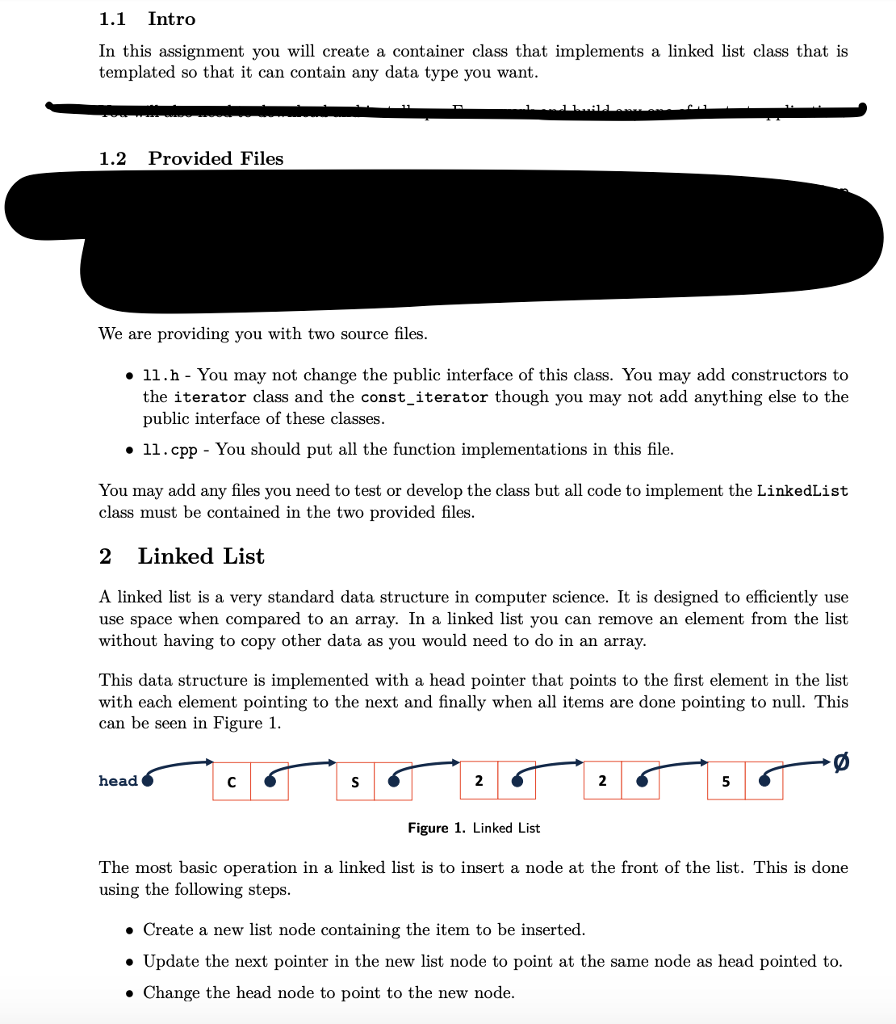
Need Help Asap Please Implement C Linked List Chegg Com
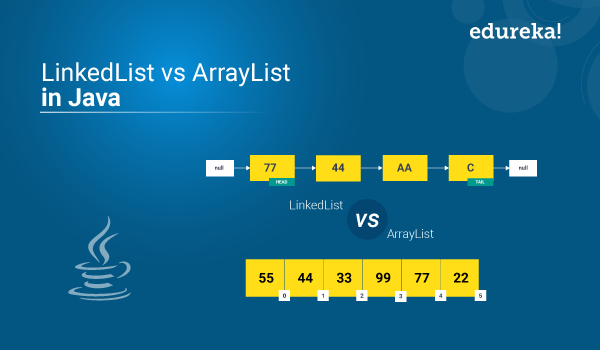
Linkedlist Vs Arraylist In Java Know The Major Differences Edureka
Iterators Programming And Data Structures 0 1 Alpha Documentation
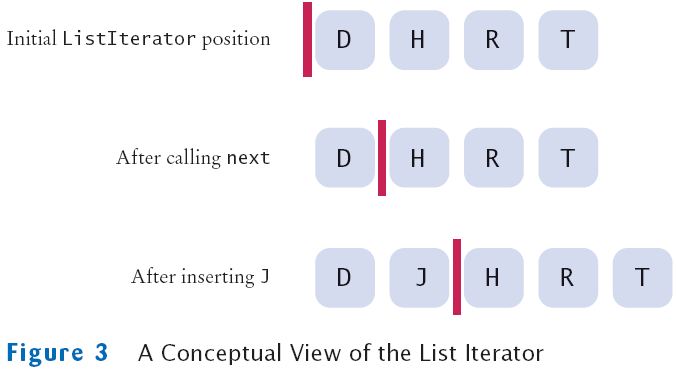
Horstmann Chapter 19
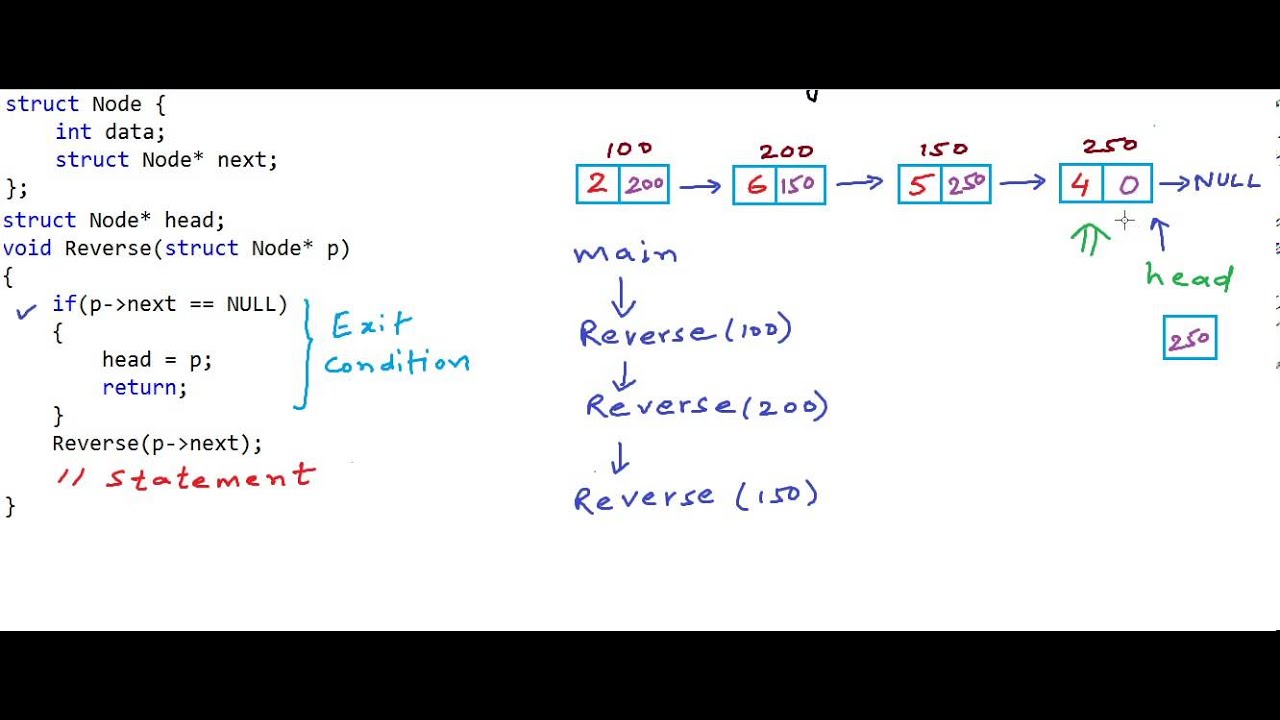
Reverse A Linked List Using Recursion Youtube

Difference Between Vector And List In C Thispointer Com
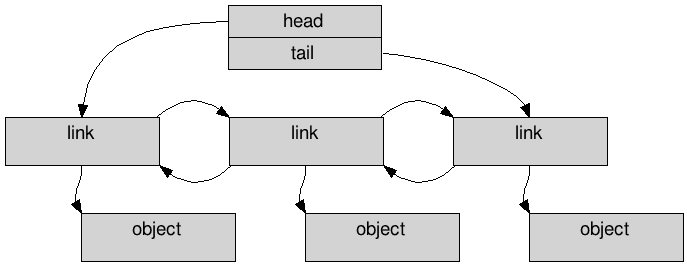
Avoiding Game Crashes Related To Linked Lists Code Of Honor
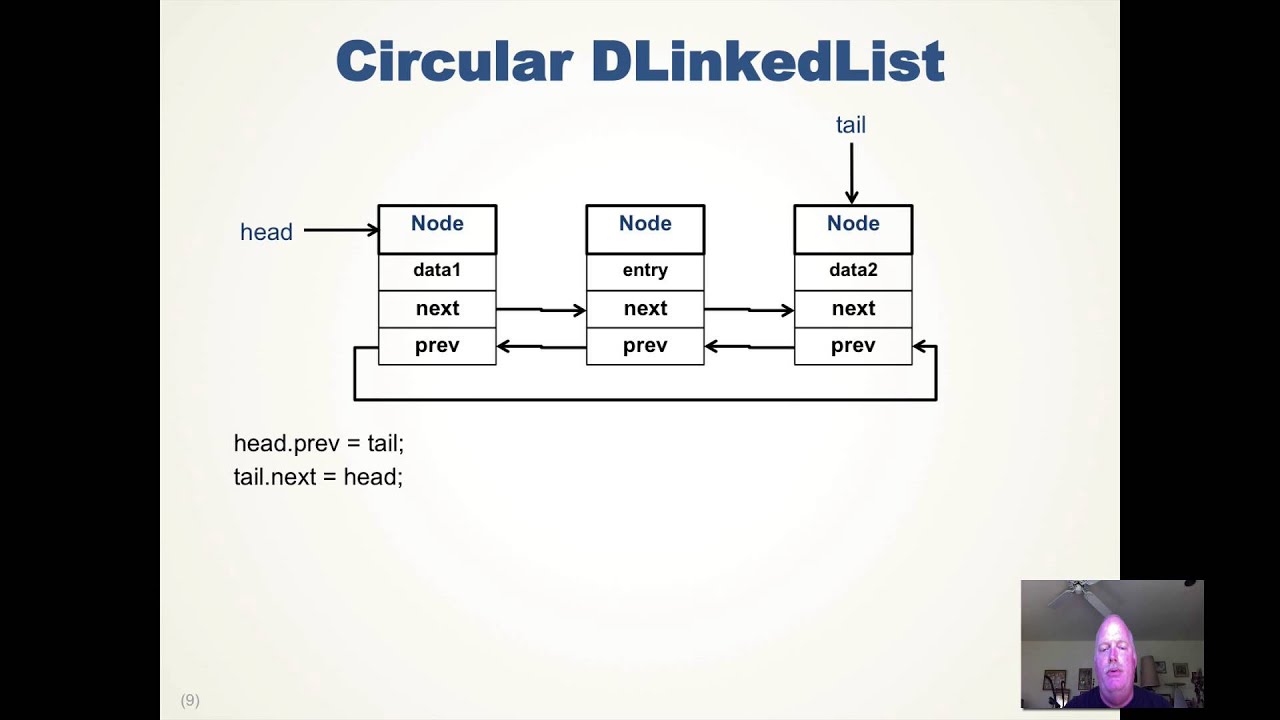
Double Linked Lists And Iterators Youtube
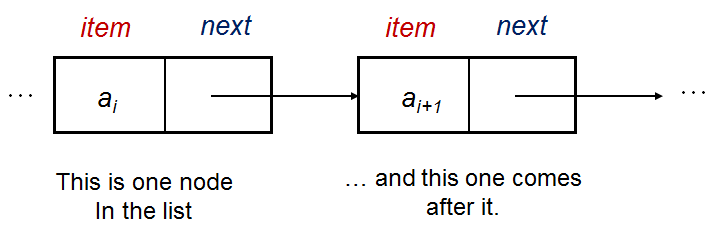
Visualgo Linked List Single Doubly Stack Queue Deque

Arraylist Vs Linkedlist Vs Vector
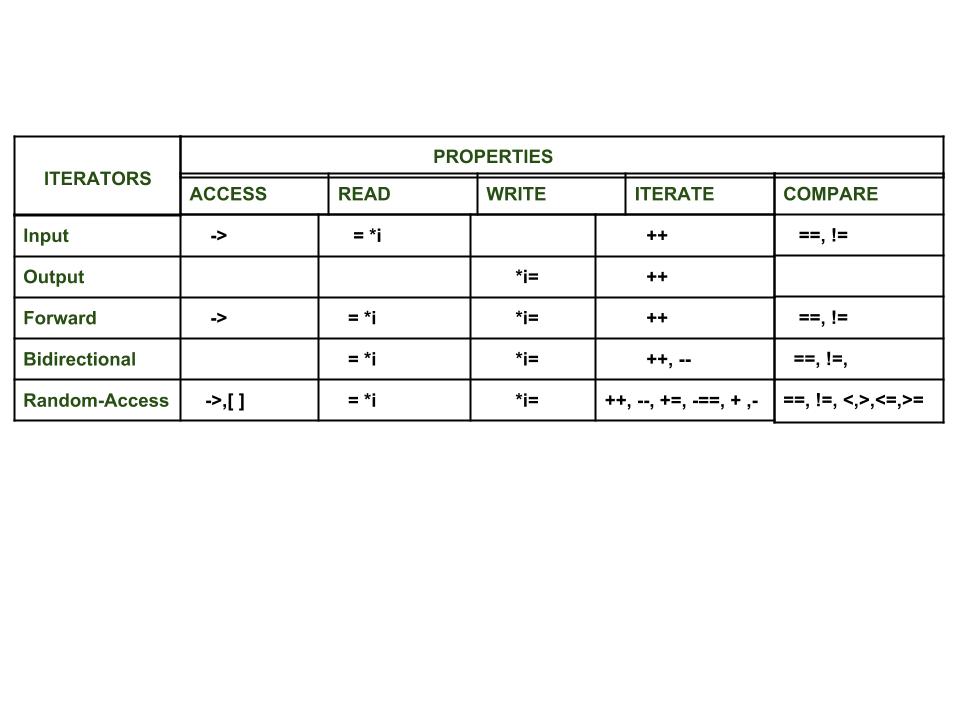
Introduction To Iterators In C Geeksforgeeks

Linked Lists And Iterative Algorithms
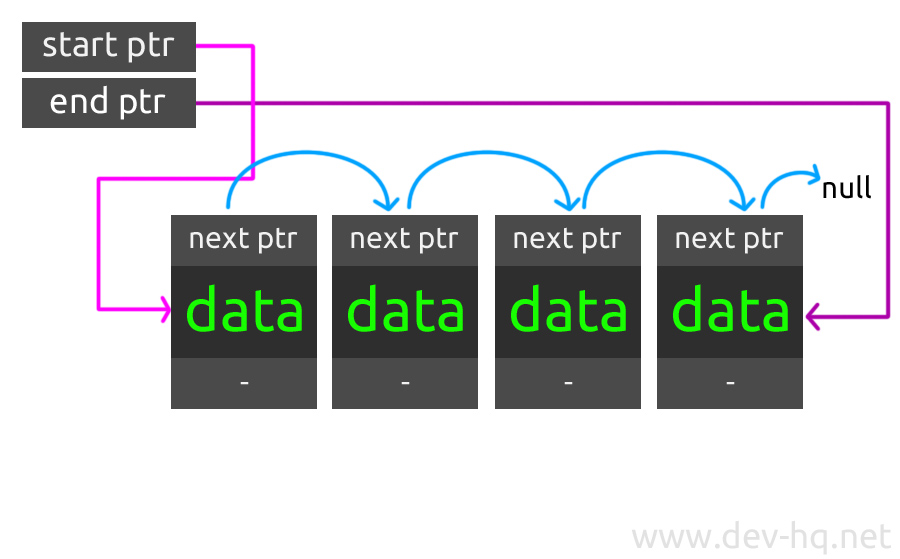
Linked Lists Dev Hq C Tutorial

C Linked Lists Are Considered Harmful Arn T They C Coding Experiments
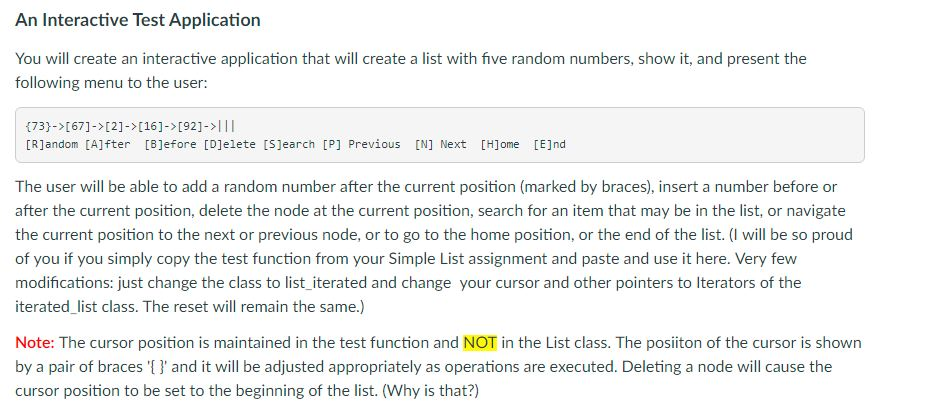
Solved C Using Linked List And Iterators Please Provid Chegg Com
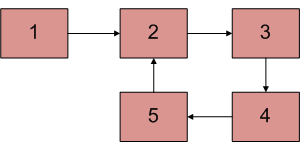
Detect And Remove Loop In A Linked List Geeksforgeeks
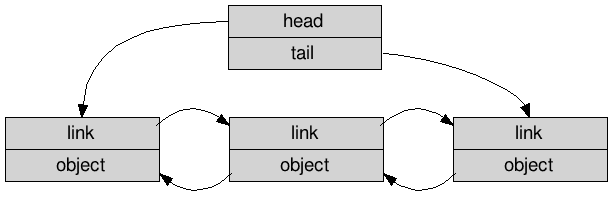
Avoiding Game Crashes Related To Linked Lists Code Of Honor

Introduction To The Linked List Data Structure And Implementation
2
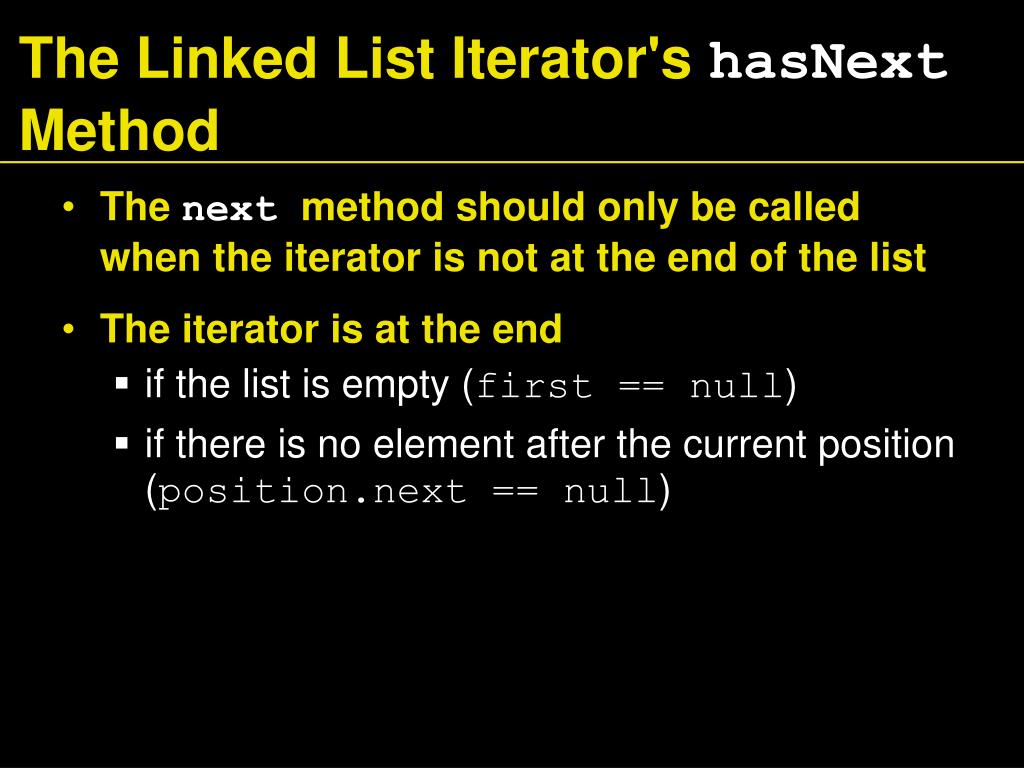
Ppt Chapter 15 An Introduction To Data Structures Powerpoint Presentation Id
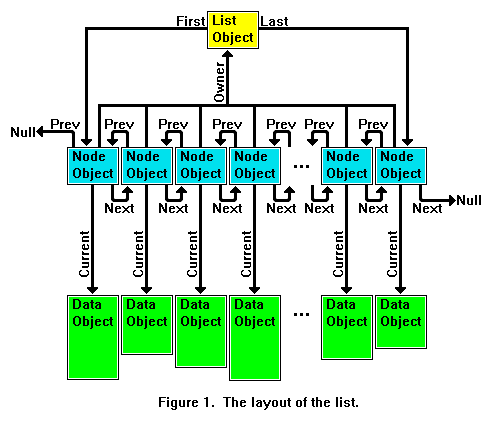
An Improved Doubly Linked List System

It C Linked List Simulation

An Introduction To Data Structures
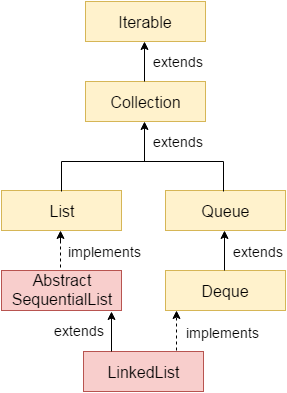
Linkedlist In Java Javatpoint
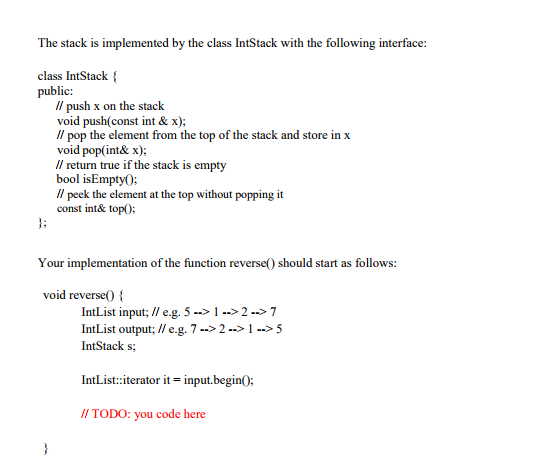
Problem 2 25 Points Write The Function Reverse0 Chegg Com

Doubly Linked List With Class Templates Part I Out4mind
How To Iterate Through A Linked List In C Quora
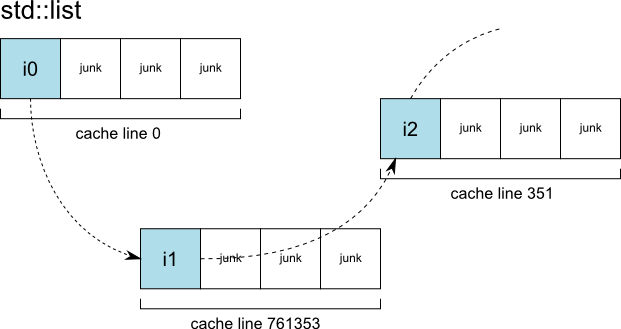
Don T Use Std List Dangling Pointers
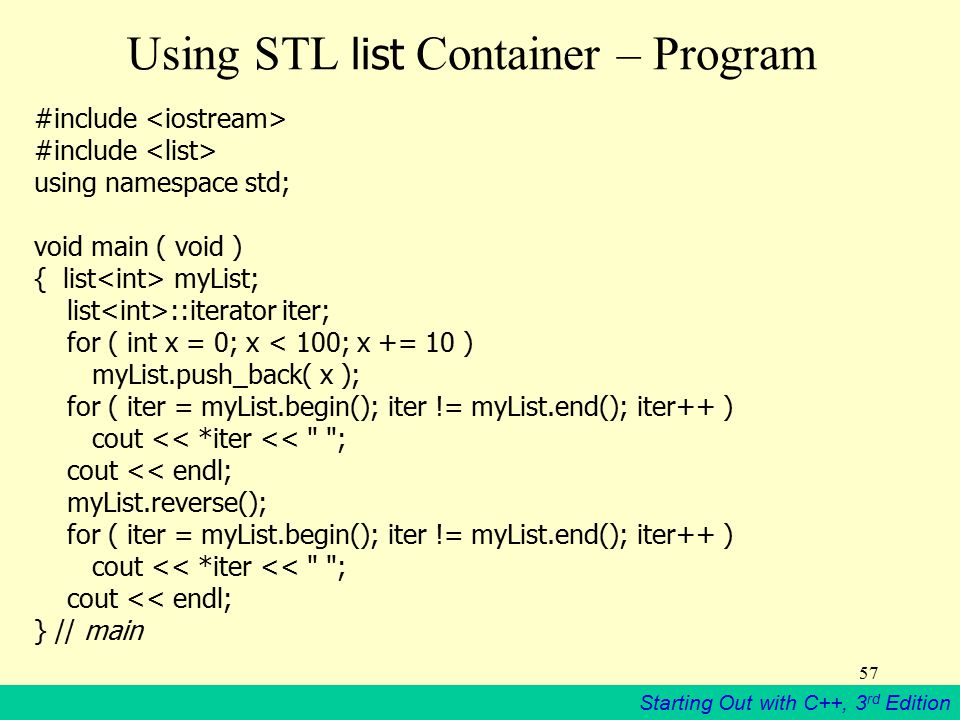
Starting Out With C 3 Rd Edition 1 Chapter 17 Linked Lists Ppt Download
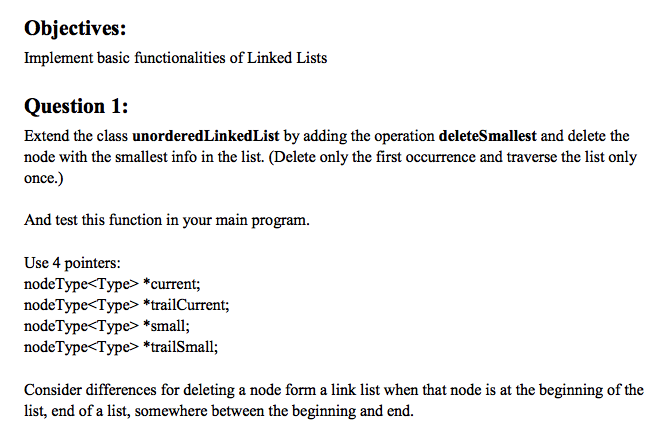
Solved C Programming Required Program Linkedlist H U Chegg Com

An Introduction To Data Structures
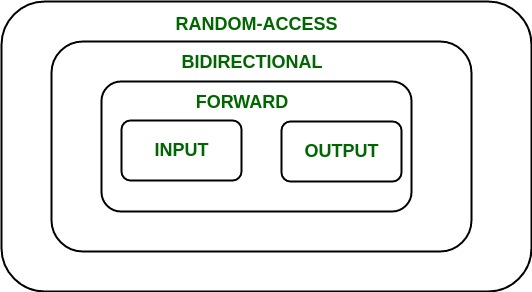
Introduction To Iterators In C Geeksforgeeks

Linked List Implementation In Abap Using Iterator Dp
Simplified C Stl Lists
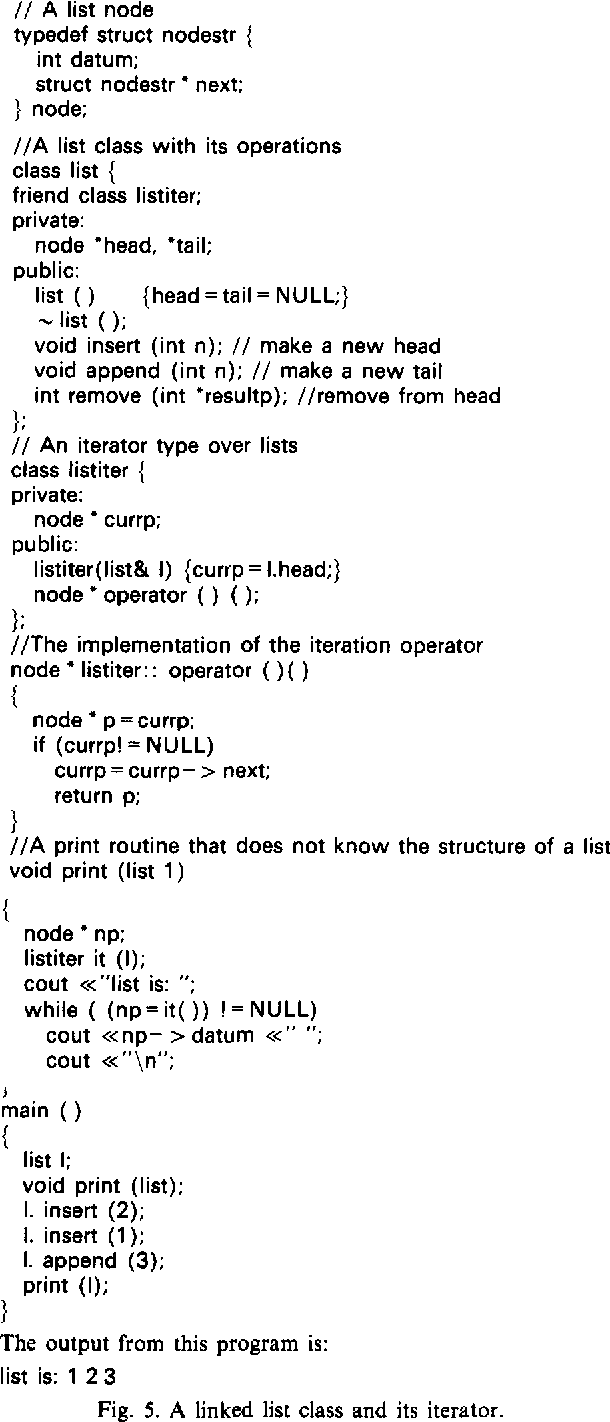
Figure 5 From C Solving C S Shortcomings Semantic Scholar
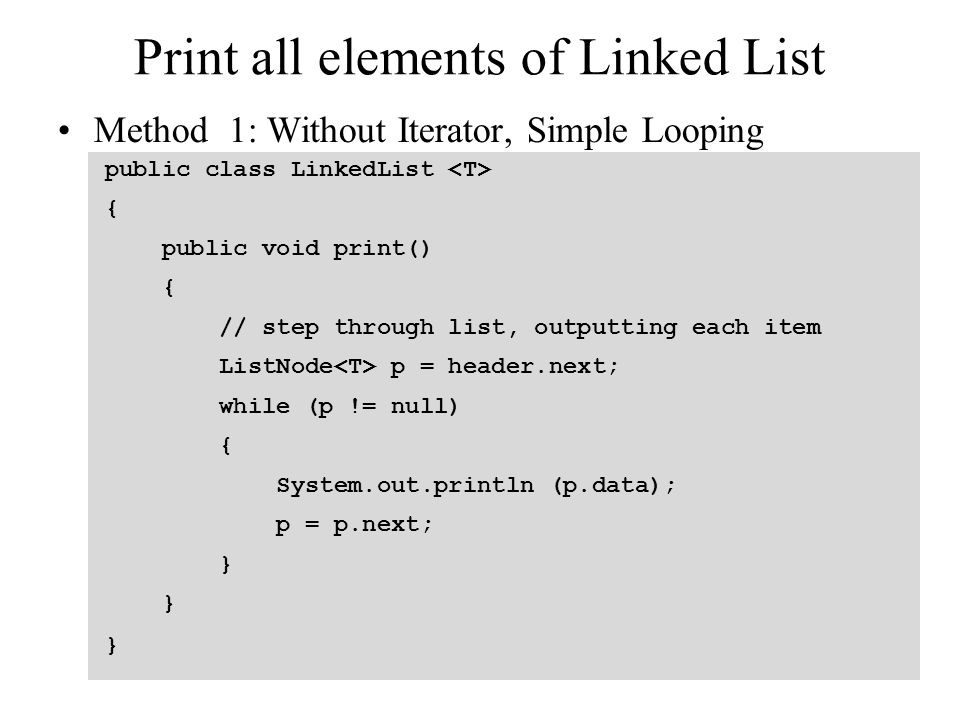
M180 Data Structures Algorithms In Java Linked Lists Arab Open University Ppt Download

Linked Lists Implementation Sequences In The C Stl

Remove Duplicate From A Linked List In C Codespeedy
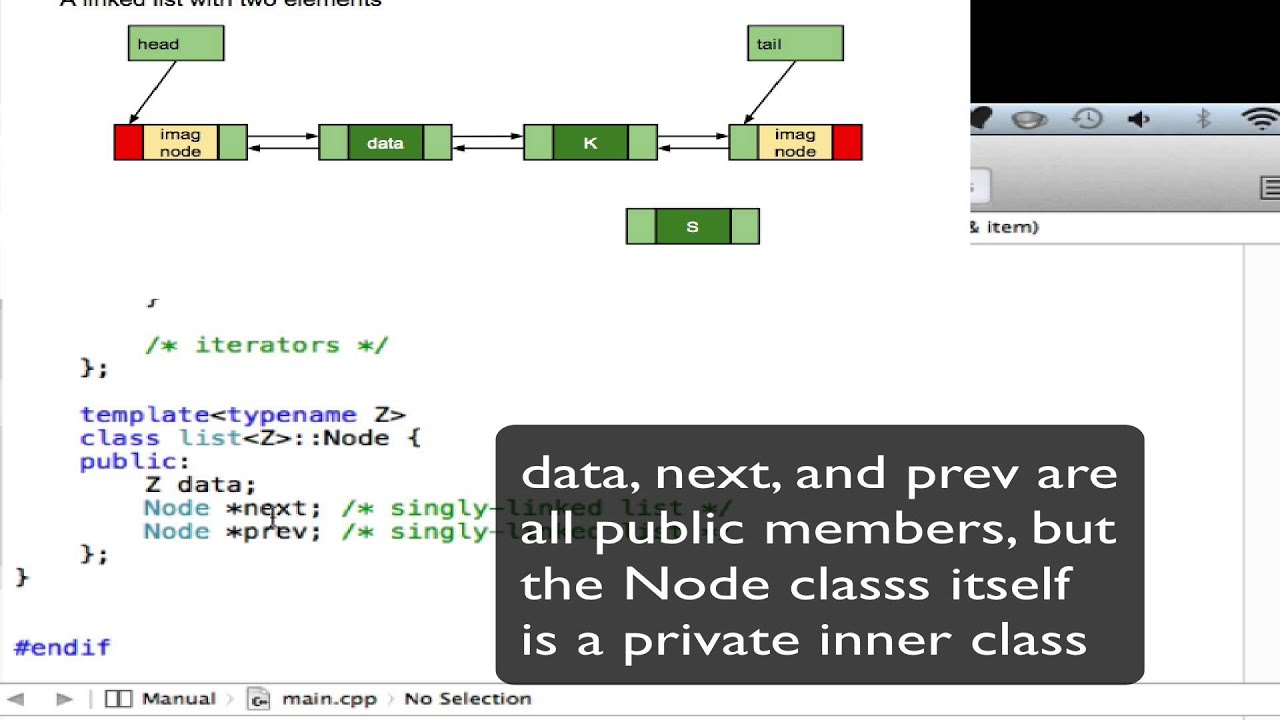
Designing C Iterators Part 3 Of 3 List Iterators Youtube
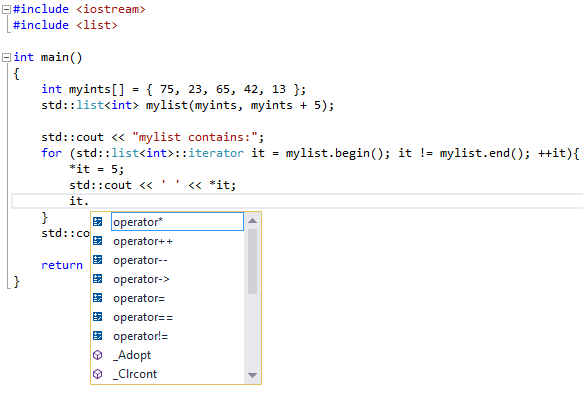
C Doubly Linked List With Iterator 矩阵柴犬 Matrixdoge
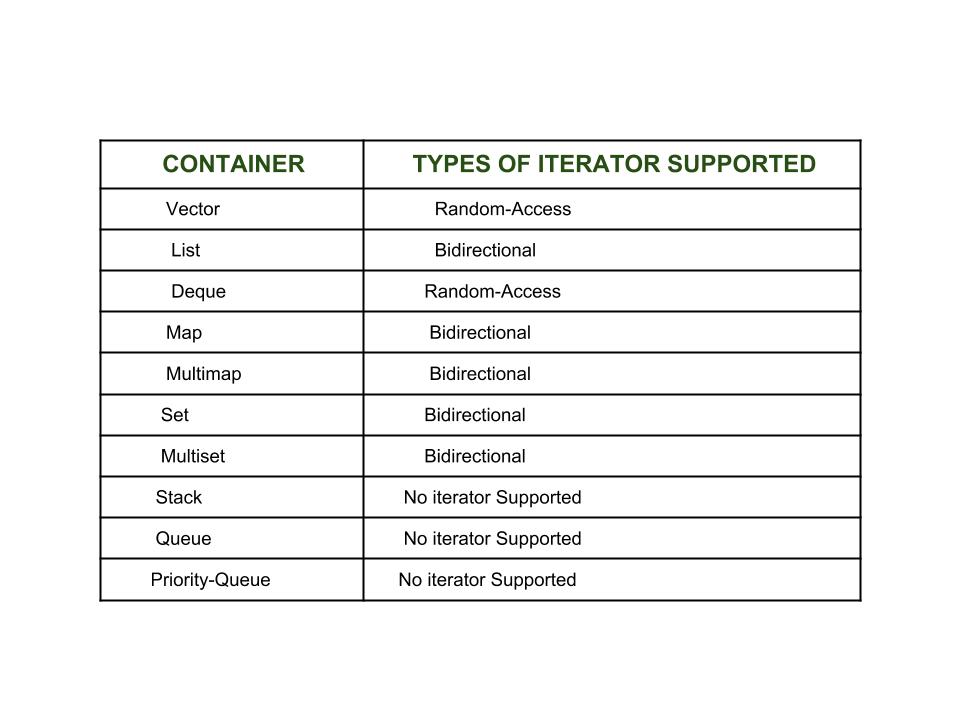
Introduction To Iterators In C Geeksforgeeks
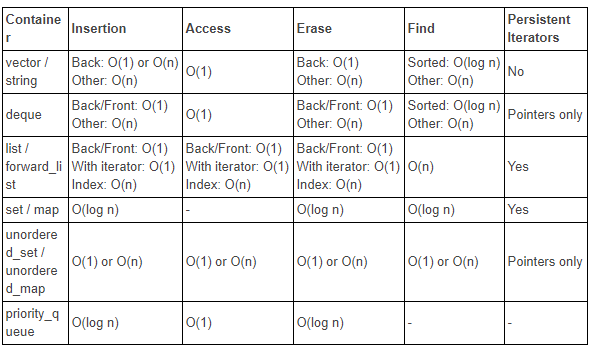
Stl Container Performance Stl Container Performance Table By Onur Uzun Medium
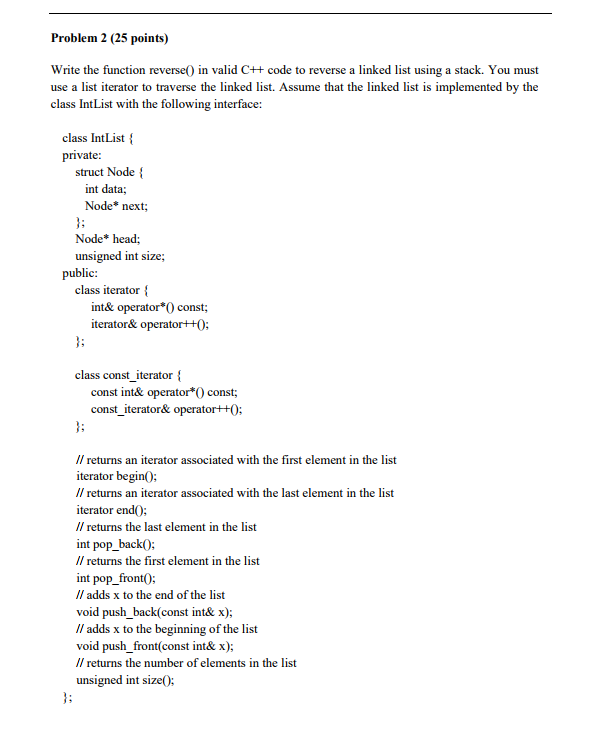
Problem 2 25 Points Write The Function Reverse0 Chegg Com
Cs 2150 02 Lists Slide Set

Java Battle Royal Linkedlist Vs Arraylist Vs Dynamicintarray Kjellkod S Blog

C Creating And Printing An Stl List Instead Of A Linked List Stack Overflow
Web Stanford Edu Class Cs107l Handouts 04 Custom Iterators Pdf

Singly Linked Lists In C Part Iii Out4mind

Object Oriented Software Engineering In Java

Computer Science In Javascript Linked List Human Who Codes

Implementation Of Doubly Linked List Code Review Stack Exchange
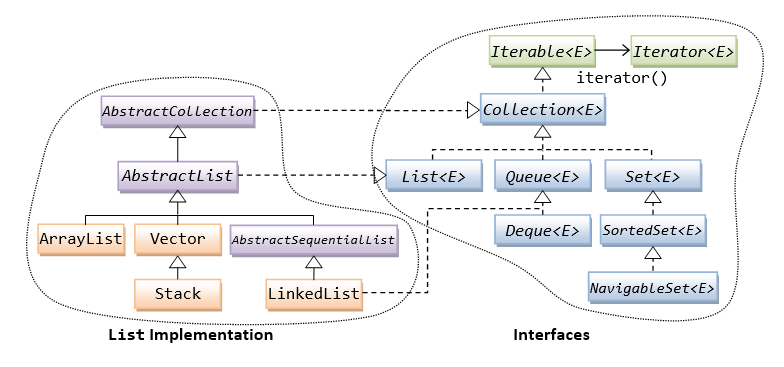
The Collection Framework Java Programming Tutorial
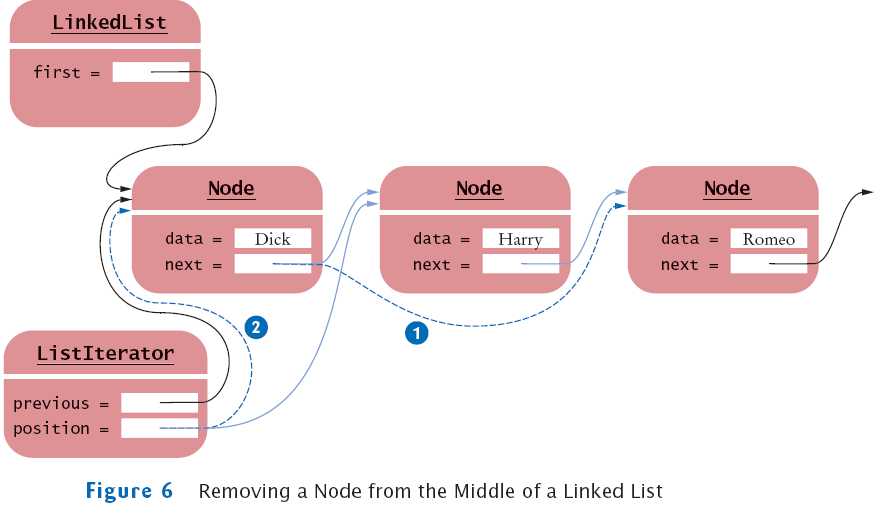
Horstmann Chapter 19

Doubly Linked List Implementation Guide Data Structures

Doubly Linked List Implementation Guide Data Structures
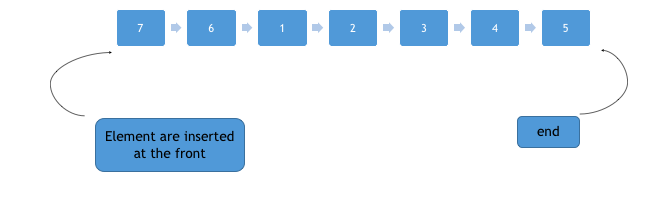
Stl List Container In C Studytonight
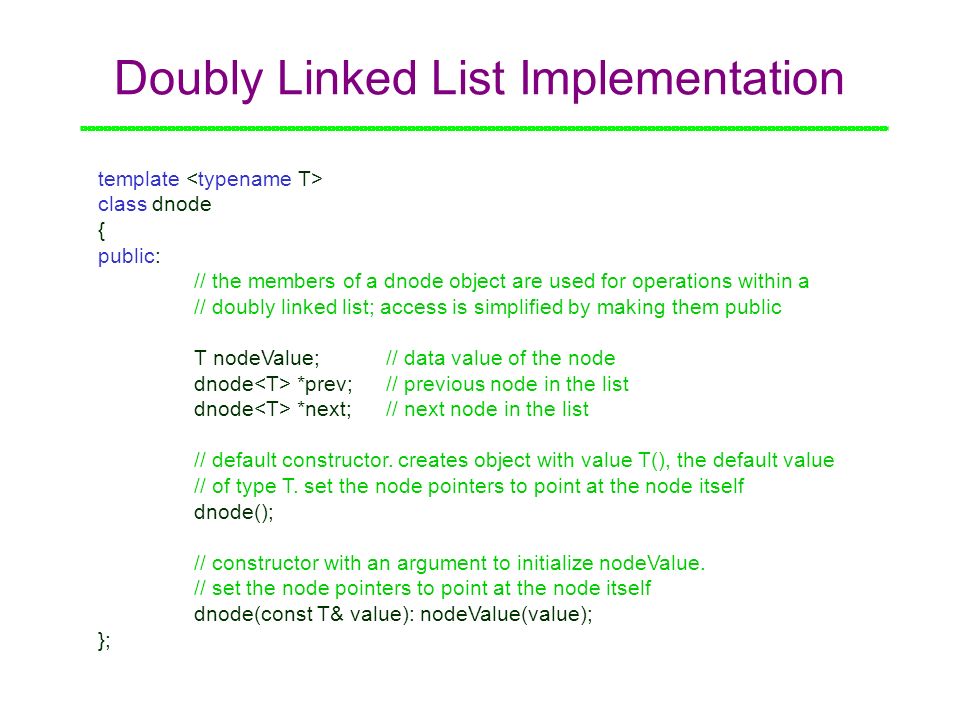
A Doubly Linked List Prevnextdata There S The Need To Access A List In Reverse Order Header Dnode Ppt Download

How To Implement A Square List Iterator Stack Overflow

Create An Empty Linkedlist By Using Listiterator Insert Several Integers Into This List When Inserting They Are Always Inserted In The Middle Of The List Programmer Sought
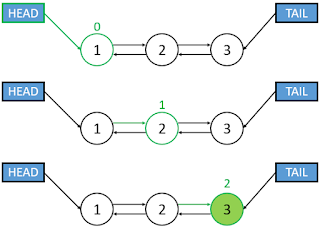
Javarevisited How To Find Middle Element Of Linked List In Java In Single Pass

How To Create A Doubly Linked List C Part 2 Youtube

A Biggish Cpp17 Linked Lists Tutorial Modern C What The Other Tutorials Don T Say Examples That You Can Try
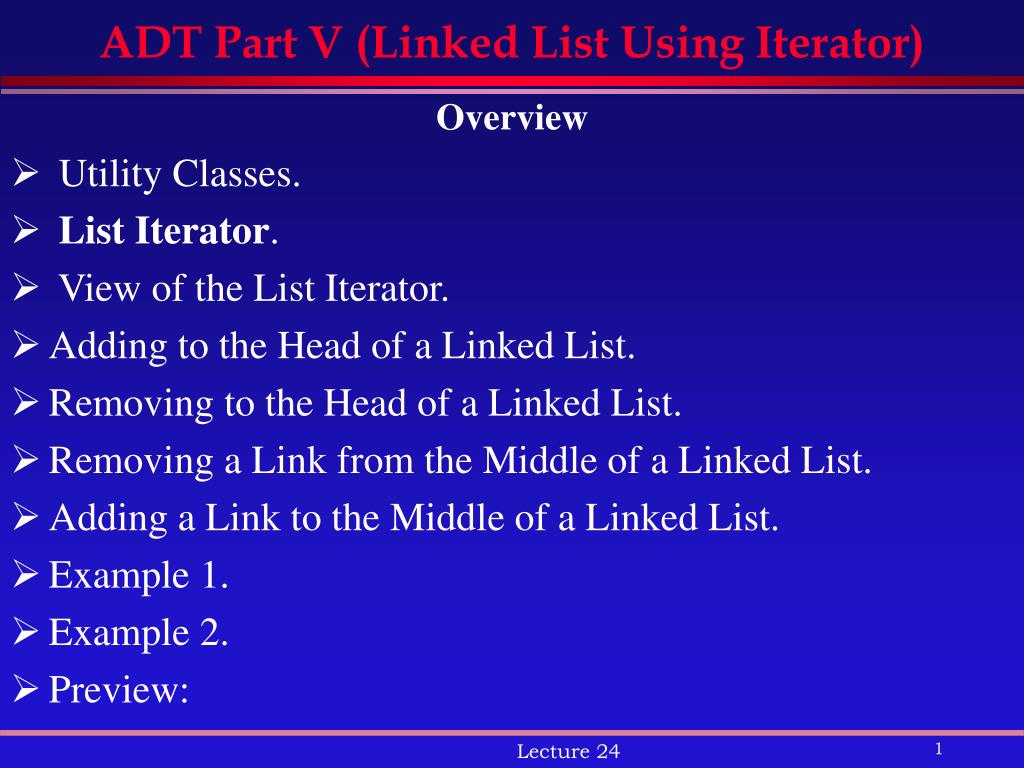
Ppt Adt Part V Linked List Using Iterator Powerpoint Presentation Free Download Id
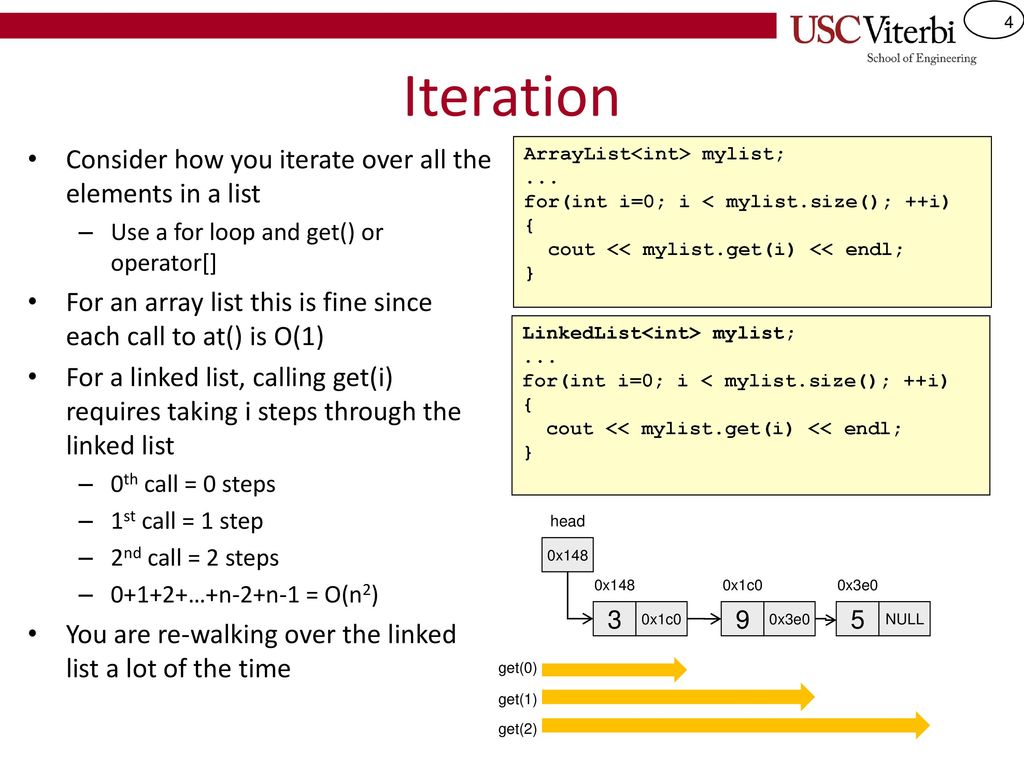
Csci 104 C Stl Iterators Maps Sets Ppt Download
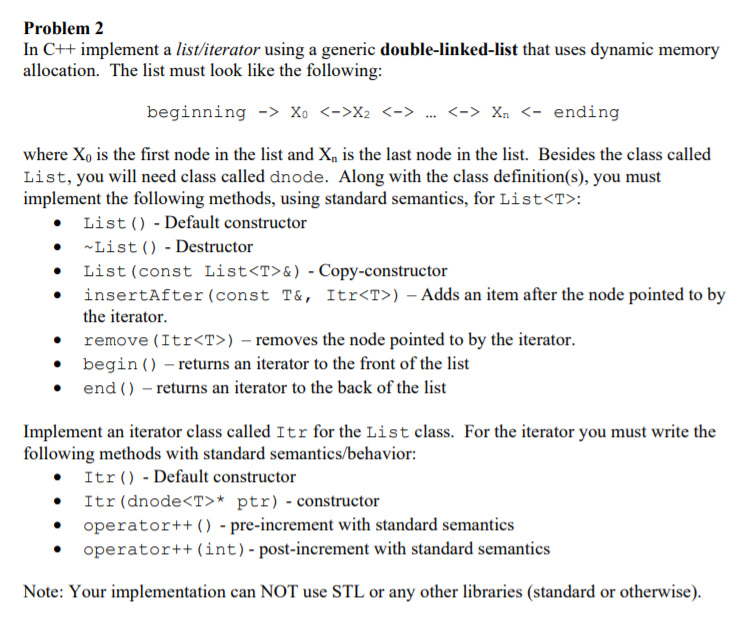
Solved In C Implement A List Iterator Using A Generic D Chegg Com

Linkedlists
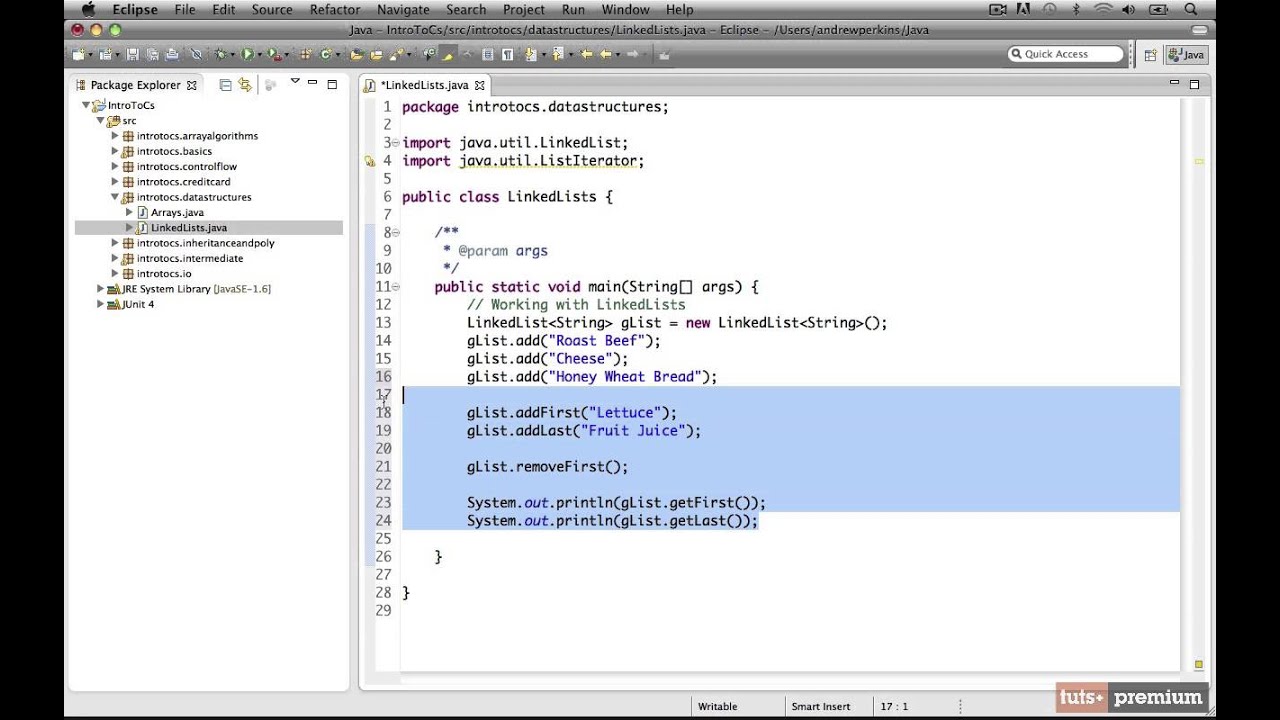
09 Linkedlist The Iterator Youtube
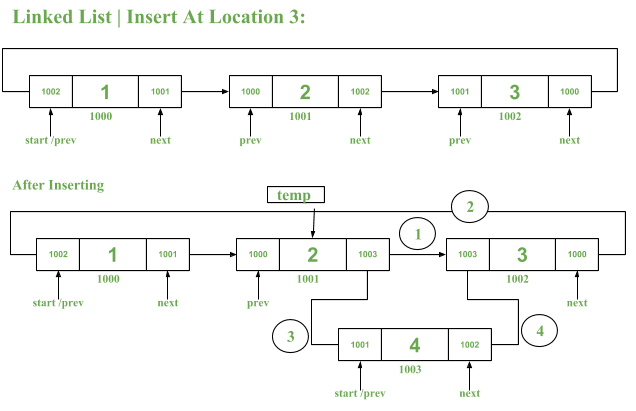
Insertion At Specific Position In A Circular Doubly Linked List Geeksforgeeks

Self Organizing Sorted Linked List In C By Vardan Grigoryan Vardanator Medium

Doubly Linked List Implementation Guide Data Structures
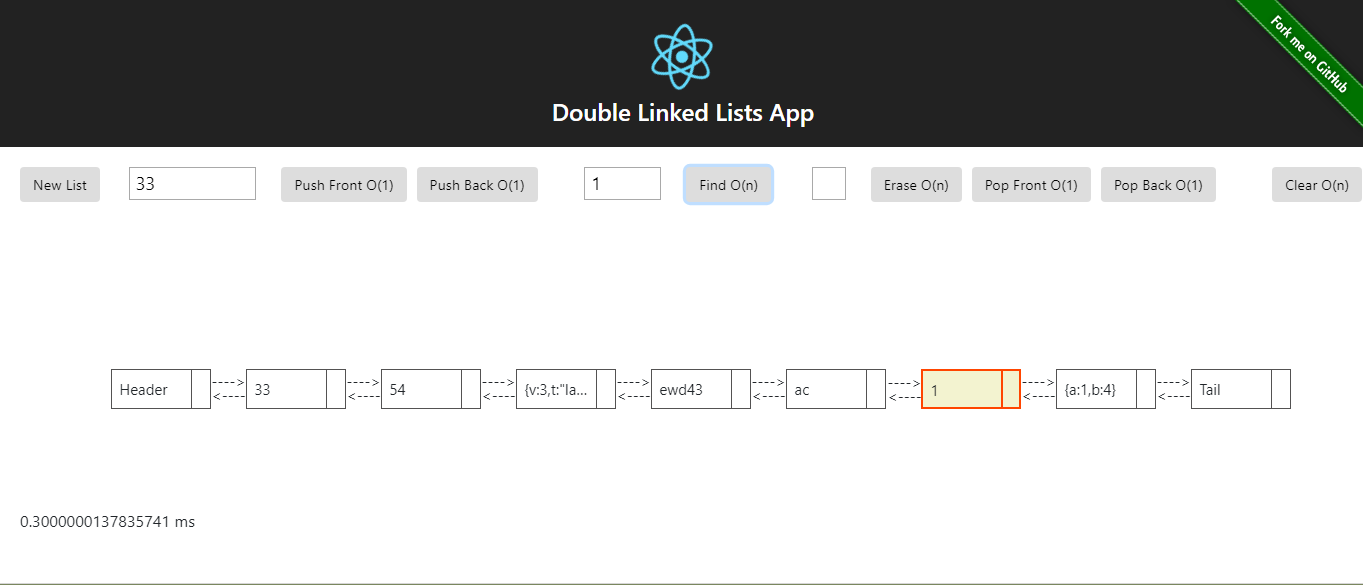
Data Structures In Js Part 2 Double Linked Lists By Oliver Alonso Itnext

A Reusable Linked List Class C C Assignment Help Online C C Project Help Homework Help
Q Tbn 3aand9gcrbfibhihr2cgljsf2lrwku9z9csz6yjt9mhas5z13qxv8jadmv Usqp Cau

Linked List In Java Linked List Implementation Java Examples
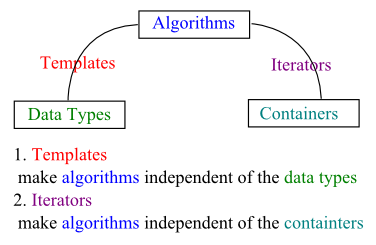
C Tutorial Stl Iii Iterators
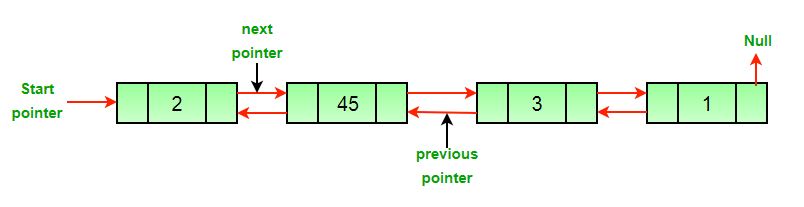
Delete A Node In A Doubly Linked List Geeksforgeeks

Number Crunching Why You Should Never Ever Ever Use Linked List In Your Code Again Kjellkod S Blog
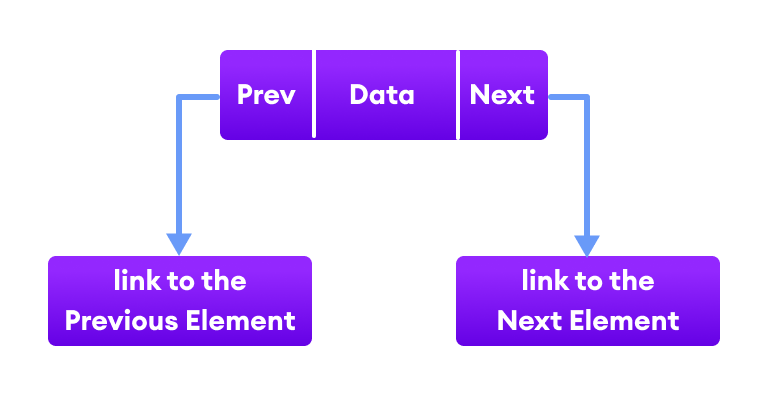
Java Linkedlist With Examples

Data Structures And Algorithm Analysis In C 4th Edition Weiss Solutions Manual By Sydney Issuu

Linkedlist 11 Iterators Youtube
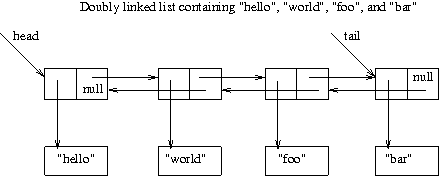
Lecture
Q Tbn 3aand9gctsoqbarh Mtup9lo5fc Gclrzluvg6kyq3r9nbrf466kbge3da Usqp Cau

Cmsc 341 Spring 1999 Section 3project 2

Help With My Custom Iterator And Linkedlist Pls Codinghelp

Performance Differences Between Arraylist And Linkedlist Stack Overflow
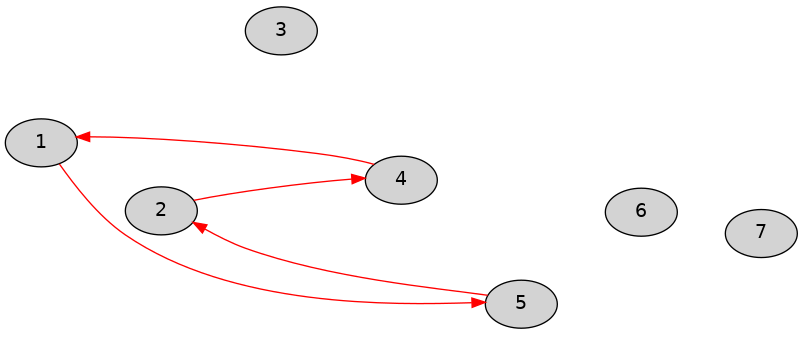
Designing A Safe Doubly Linked List With C Like Iterators In Rust Parts 1 And 2 Soft Silverwind S Blog

Difference Between Arraylist Vs Linkedlist Updated

Cs2 Uml Object Models

Linked List In Java Linked List Implementation Java Examples

Iterators For Custom Datastructure Stack Overflow
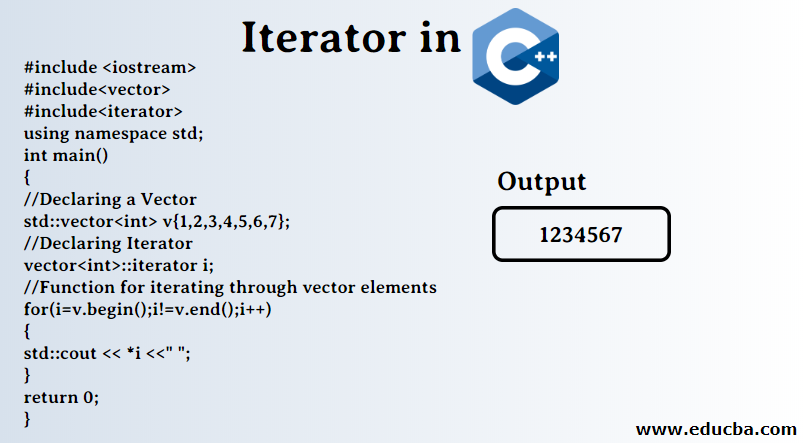
Iterator In C Operations Categories With Advantage And Disadvantage

Linkedlists

Linked List Linked Lists In Python An Introduction Real Python
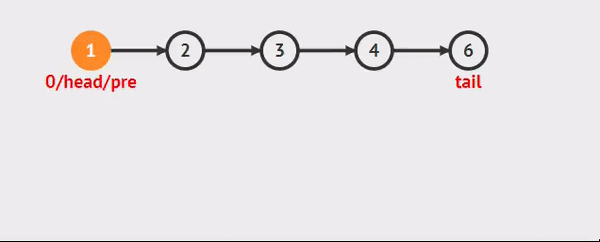
Q Tbn 3aand9gcr35 Lrbfcpcamzergjh5wglcbg9k2vz Hoew Usqp Cau
How To Implement A Doubly Linked List In C Quora